Hello everyone! In my previous post I had my first try of chatGPT [1], a revolutionary conversation bot that answers questions in a human-like dialogue. I shared my thoughts on chatGPT, its technology, and its possible societal implications. I also asked it to write a Christmas poem for me, which was pretty good! In this post, I am going to go deeper into using chatGPT. I will write Python code with the help of chatGPT, and it will be awesome!
Coding before chatGPT
I started coding before the Internet age. When I was 13, I wrote my first Basic program with some machine code to operate with the graphic card memory. It was a flight simulation game on a ZX-Spectrum computer. That involved loads of book reading and also looking into the documentation. Several years later, the Internet started, but the primary source of coding-related information was primarily available in books. However, most of the learning was done by doing, experimenting with code, and trying different techniques.
Nowadays, The Internet, search engines, and professional forums give us tremendous support to learn together, share, and receive coding tips. There are so many tutorials on Medium and StackOverflow that the coding process is much easier! All the information is at hand, and we have the luxury of borderless communication and exchanging ideas.
Today, we are beginning something fantastic in programing. This is when machines can help us in the coding process, and we can learn much faster. Let’s see how we can learn coding with chatGPT, for example, building a simple neural network in Python.
chatGPT and its technology
The chatGPT demonstrates the usage GPT-3 language model trained on a sizeable dataset. As I have pointed out in my previous post “chatGPT Wrote me a Christmas Poem”, the exact architecture of this model has yet to be disclosed. Nevertheless, it gives outstanding results. It also generates Python code “on the fly,” as we will see next.
Can chatGPT pass the Turing test, which tests that a machine can show human-like intelligence? In my opinion, chatGPT gives “too perfect responses” comparable to a well-designed customer service providing information very quickly. I have asked chatGPT about it.
Elena: Can you pass the Turing test?
chatGPT: As an AI language model, I am not able to interact with people in the same way that a human can. I don't have senses or the ability to perceive the world in the same way that humans do, so I am unable to pass the Turing test in the traditional sense. However, I am able to engage in conversation and respond to questions and prompts in a way that may seem similar to a human. My responses are generated based on patterns and information that I have learned from a vast amount of text data, but I do not have personal experiences or emotions like a human does.
Learning to Code with chatGPT
Even though chaGPT is not a coding coach, we can use it to learn to program while conversing in a human-like chat.
Elena: Hi! Can you help me to write a Python code?
chatGPT:
Sure! I'd be happy to help you write Python code. Before we get started, it would be helpful if you could tell me a little bit more about what you're trying to do. Do you have a specific task in mind that you'd like to accomplish with your code, or are you just looking for general guidance on how to get started with Python programming?
Elena: I am interested to learn how Neural Networks work. To start, I want to code a neuron in Python. The neuron will have N inputs, an activation function, and one output. I need to figure out where to start. Please help!
What happened next was mind-blowing! I have seen how the chatGPT writes me a well-explained and commented Python code of the Neuron class with everything I asked for. See the screenshot with excellent syntax highlighting and an explanation of the code! Simply awesome!
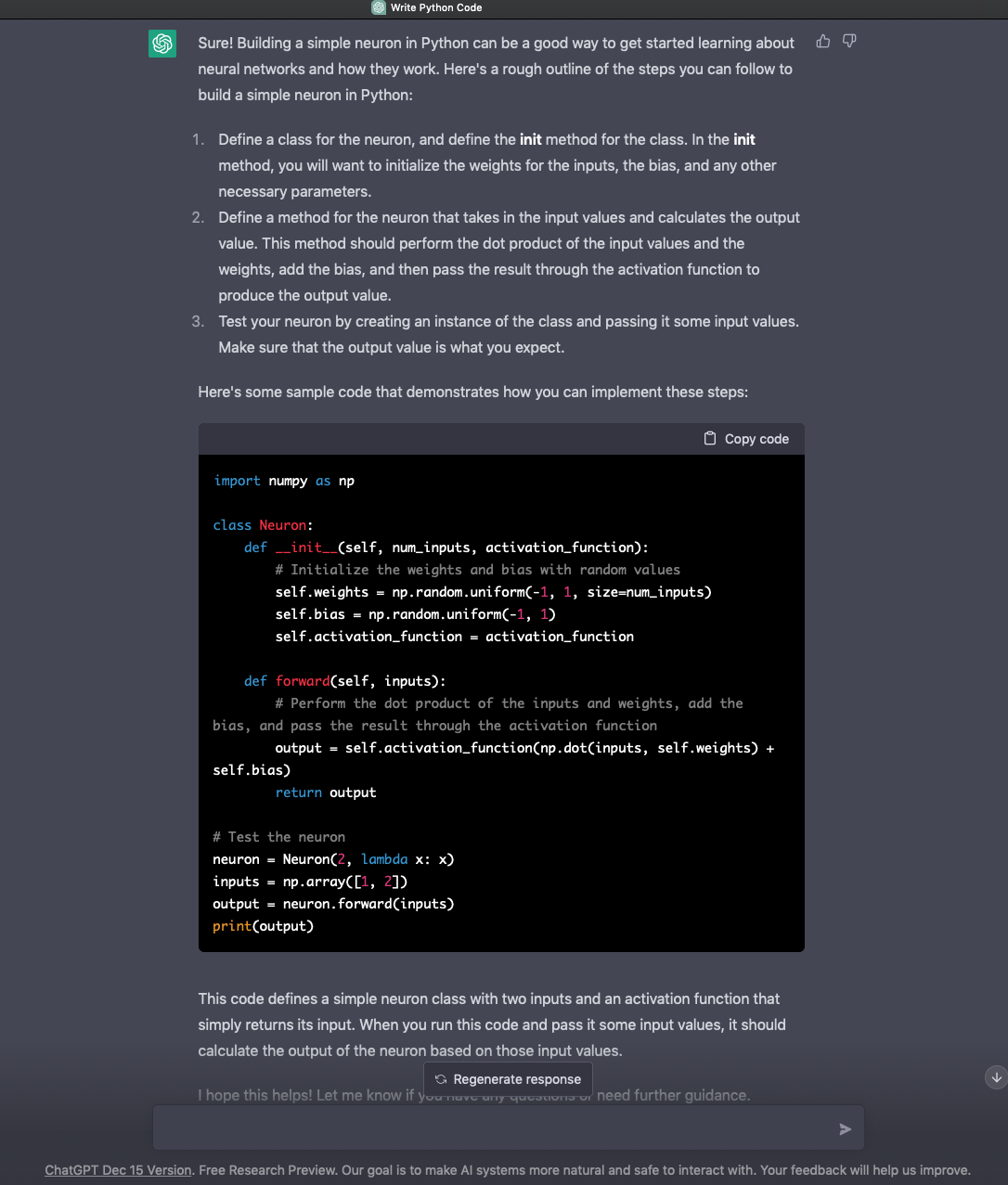
A Neuron Class coded by chatGPT
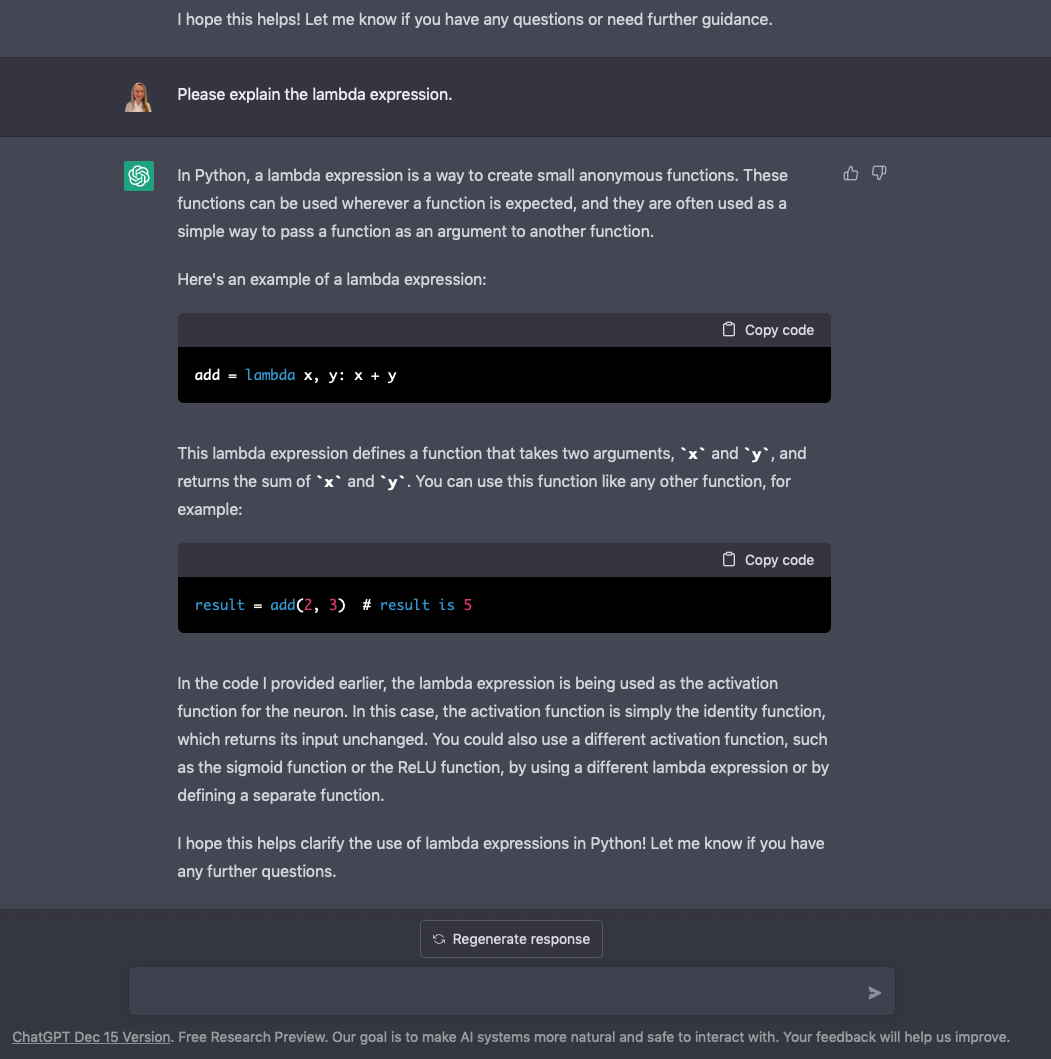
A Python lambda expression explained by chatGPT
However, I wanted to improve the neuron code further with a ReLU activation function. If you are interested, refer to my introductory post “Artificial Neural Networks” explaining the activation functions.
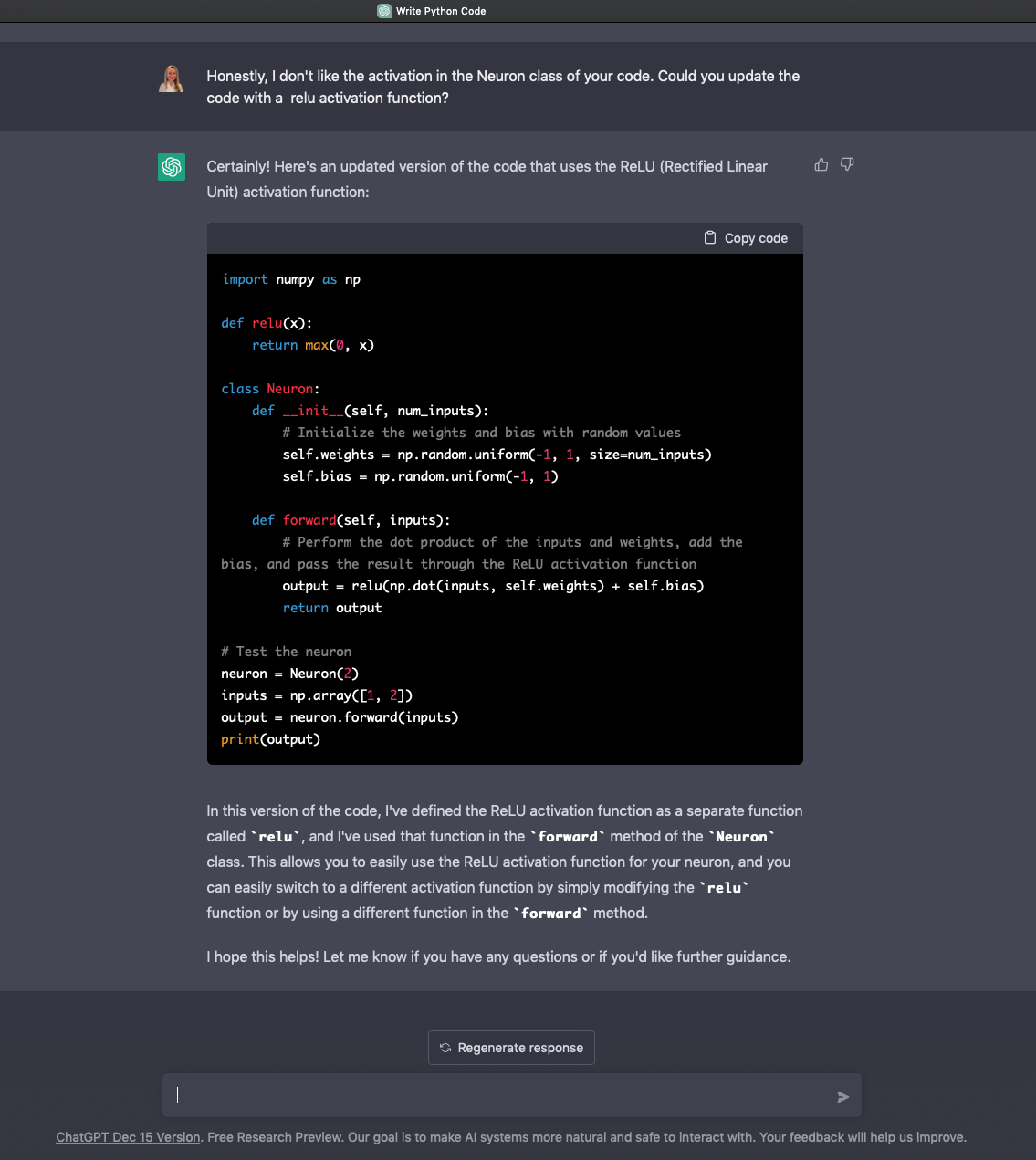
Neuron class with ReLU activation function all coded by chatGPT
Elena: Please add a tanh activation function to choose between tanh and relu activation when initializing the Neuron class.
chatGPT:
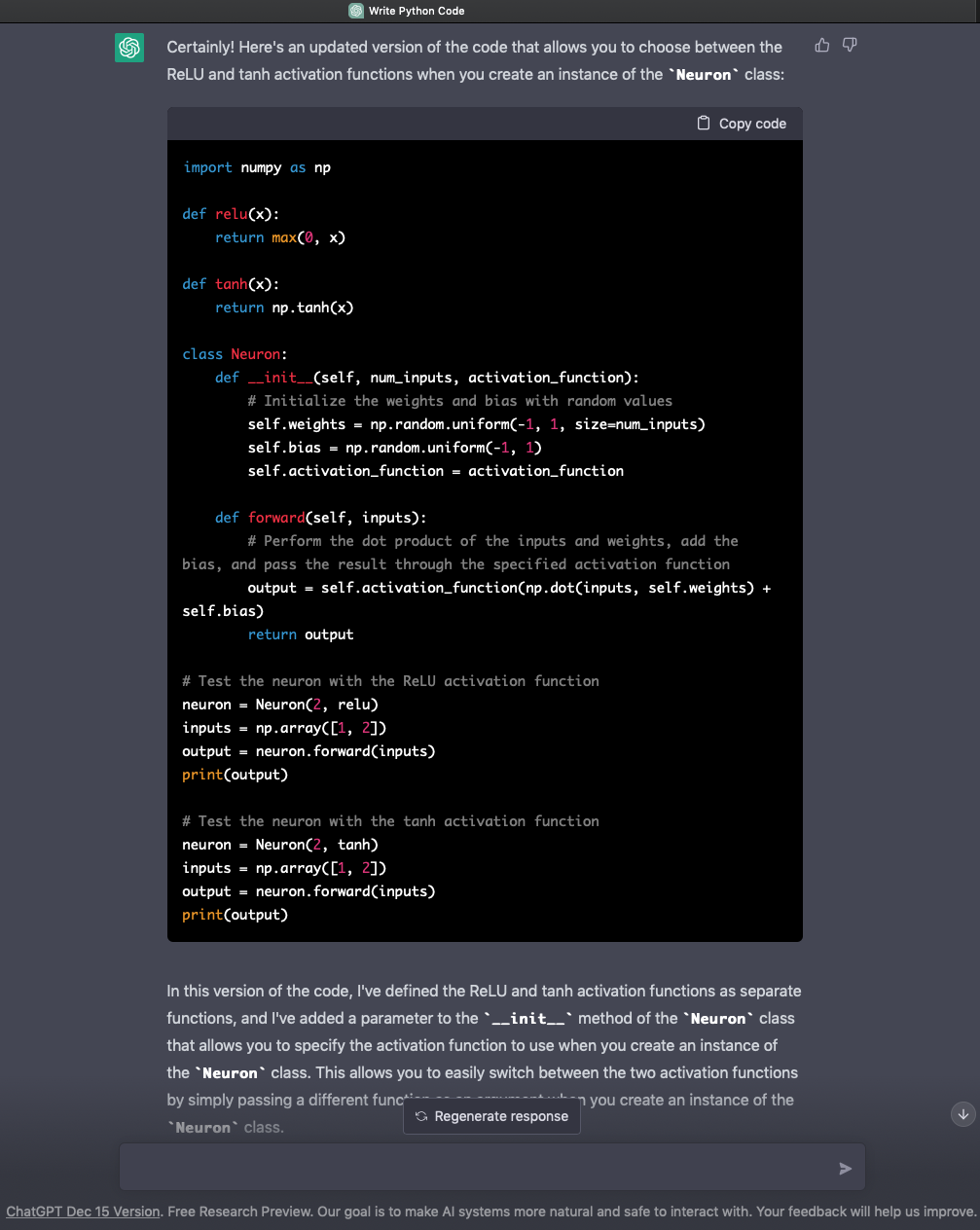
Neuron class (updated) with ReLU and TANH activation functions all coded by chatGPT
As usual, for me, that was not enough! I desperately needed to create a complete artificial neural network code.
Elena: I have one neuron. How do we connect multiple neutrons in an artificial neural network? Code me an artificial neural network using the last Neuron class, please.
chatGPT: To create an artificial neural network (ANN) using the Neuron class that I provided earlier, you can define a new class for the ANN that contains multiple instances of the Neuron class. Here's an example of how you might do this:
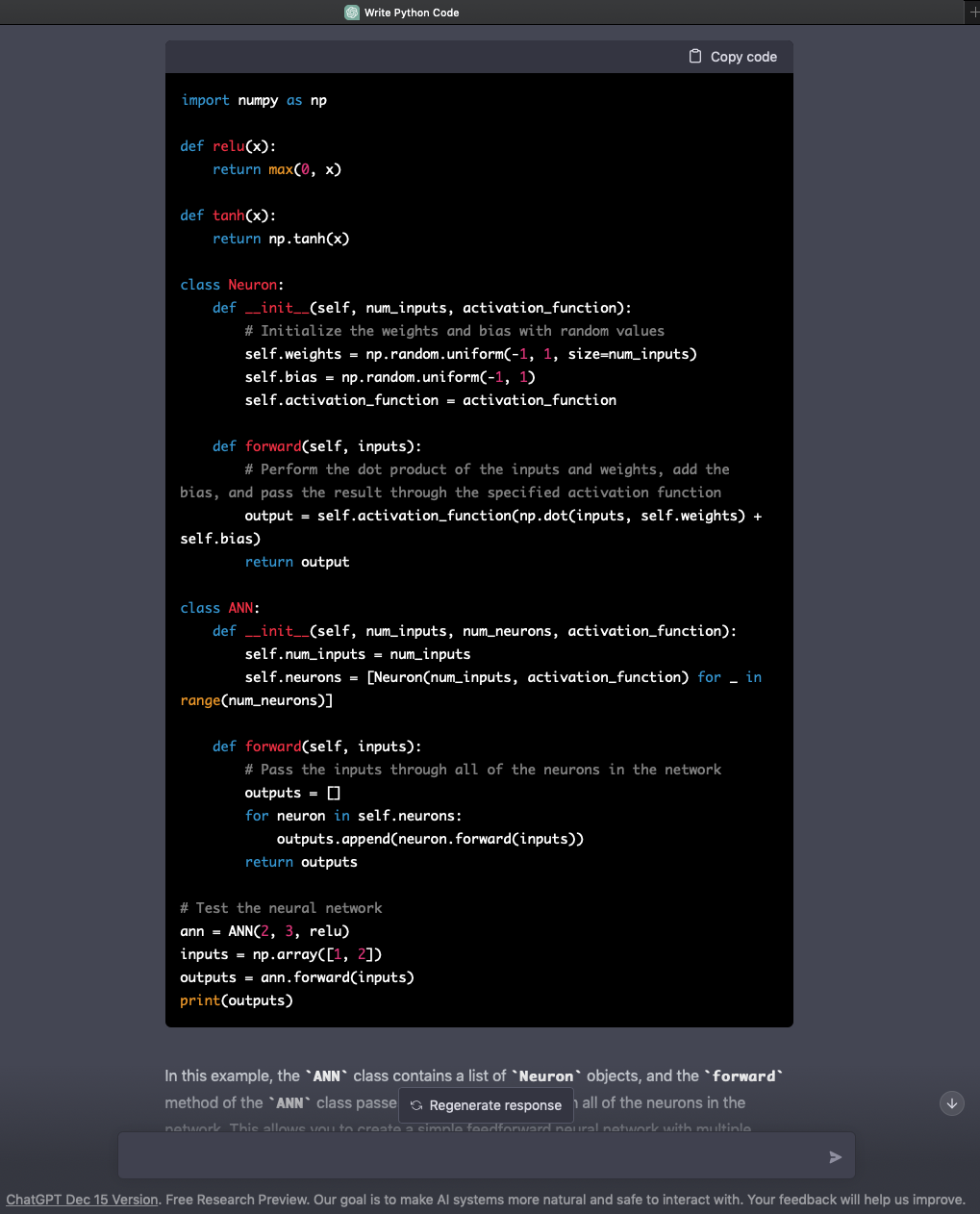
A complete ANN using Neuron class (updated) with ReLU and TANH activation functions all coded by chatGPT
chatGPT: In this example, the ANN class contains a list of Neuron objects, and the forward method of the ANN class passes the input values through all of the neurons in the network. This allows you to create a simple feedforward neural network with multiple neurons. I hope this helps! Let me know if you have any questions or if you'd like further guidance.
Elena: Sorry, I am a bit picky! I don't like the random weights. Update the latest code to find the best weights in training.
chatGPT: Certainly! Here's an updated version of the code that allows you to train the neural network by adjusting the weights of the neurons:
import numpy as np def relu(x): return max(0, x) def tanh(x): return np.tanh(x) class Neuron: def __init__(self, num_inputs, activation_function): # Initialize the weights and bias with random values self.weights = np.random.uniform(-1, 1, size=num_inputs) self.bias = np.random.uniform(-1, 1) self.activation_function = activation_function def forward(self, inputs): # Perform the dot product of the inputs and weights, add the bias, and pass the result through the specified activation function output = self.activation_function(np.dot(inputs, self.weights) + self.bias) return output class ANN: def __init__(self, num_inputs, num_neurons, activation_function): self.num_inputs = num_inputs self.neurons = [Neuron(num_inputs, activation_function) for _ in range(num_neurons)] def forward(self, inputs): # Pass the inputs through all of the neurons in the network outputs = [] for neuron in self.neurons: outputs.append(neuron.forward(inputs)) return outputs def train(self, inputs, labels, learning_rate): # Calculate the output of the network for the given inputs outputs = self.forward(inputs) # Calculate the error for each output errors = [output - label for output, label in zip(outputs, labels)] # Adjust the weights and bias of each neuron based on the error and the learning rate for i, neuron in enumerate(self.neurons): neuron.weights -= learning_rate * errors[i] * inputs neuron.bias -= learning_rate * errors[i] # Test the neural network ann = ANN(2, 3, relu) inputs = np.array([1, 2]) labels = np.array([0, 1, 0]) ann.train(inputs, labels, 0.1)
chatGPT: In this version of the code, I've added a train method to the ANN class that takes in the input values, the corresponding labels, and a learning rate. The train method calculates the output of the network for the given inputs, and then calculates the error for each output based on the difference between the output and the corresponding label. It then adjusts the weights and bias of each neuron based on the error and the learning rate. This allows you to train the neural network by adjusting the weights of the neurons in an attempt to minimize the error between the output and the labels. I hope this helps! Let me know if you have any questions or if you'd like further guidance.
Test the neural network
Elena: Thanks! Add me a test method.
chatGPT: Certainly! Here's an updated version of the code that includes a test method for the neural network:
import numpy as np def relu(x): return max(0, x) def tanh(x): return np.tanh(x) class Neuron: def __init__(self, num_inputs, activation_function): # Initialize the weights and bias with random values self.weights = np.random.uniform(-1, 1, size=num_inputs) self.bias = np.random.uniform(-1, 1) self.activation_function = activation_function def forward(self, inputs): # Perform the dot product of the inputs and weights, add the bias, and pass the result through the specified activation function output = self.activation_function(np.dot(inputs, self.weights) + self.bias) return output class ANN: def __init__(self, num_inputs, num_neurons, activation_function): self.num_inputs = num_inputs self.neurons = [Neuron(num_inputs, activation_function) for _ in range(num_neurons)] def forward(self, inputs): # Pass the inputs through all of the neurons in the network outputs = [] for neuron in self.neurons: outputs.append(neuron.forward(inputs)) return outputs def train(self, inputs, labels, learning_rate): # Calculate the output of the network for the given inputs outputs = self.forward(inputs) # Calculate the error for each output errors = [output - label for output, label in zip(outputs, labels)] # Adjust the weights and bias of each neuron based on the error and the learning rate for i, neuron in enumerate(self.neurons): neuron.weights -= learning_rate * errors[i] * inputs neuron.bias -= learning_rate * errors[i] def test(self, inputs, labels): # Calculate the output of the network for the given inputs outputs = self.forward(inputs) # Calculate the error for each output errors = [output - label for output, label in zip(outputs, labels)] # Calculate the mean squared error (MSE) mse = sum([error**2 for error in errors]) / len(errors) return mse # Test the neural network ann = ANN(2, 3, relu) inputs = np.array([1, 2]) labels = np.array([0, 1, 0]) ann.train(inputs, labels, 0.1) mse = ann.test(inputs, labels) print(mse)
0.6538622919119595
chatGPT: In this version of the code, I've added a test method to the ANN class that takes in the input values and the corresponding labels, and calculates the mean squared error (MSE) between the output of the network and the labels. The MSE is a common measure of the accuracy of the network, and it is calculated by taking
Elena: Please create a function to plot MSE regarding the learning rate.
chatGPT: Certainly! Here's an example of how you might create a function to plot the MSE of a neural network as a function of the learning rate:
At this point, chatGPT got stacked several times. Hopefully, it will finish the function later. It could be that it needed to rest or have a cup of coffee after slave coding for me.
Interestingly, I adjusted the learning rate to 0.5 when calling the train function, and the MSE improved. Try it yourself and let me know what you think.
Coding outcomes
That’s it! We exploited the chatGPT to write a Neron class with a ReLU/Tanh activation function and
created an artificial neural network. Unfortunately, it did not complete the function
for plotting the MSE error against the learning rate. Indeed, we can finish this function ourselves.
To sum up my code challenge for chatGPT, I observed the knowledge of what we really expect as the end result of coding. We need to know the usual practise and what parameters or functions are generally used. I have to request chatGPT to write me specific activation functions.
What’s next?
Conversation bots will be part of our daily life in customer service or information retrieval tasks. That will surely mean some support personnel might become out of a job or need to be retrained. The future is here, and we need to be prepared for a more exciting but challenging life wherein AI is part of our daily lives. The evolution of AI will certainly impact our lives and businesses.
The future of programming is bright. Tools such as chatGPT or other automatic code completion tools such as Tabnine or GitHub co-pilot will make coding more efficient to learn and do. Eventually, we might expect a total automation of coding and software creation. Now, AI helps us to automate tedious tasks while also exploring our creativity.
I don’t seek to make our lives easy-going or lazy. I want AI to assist us in repetitive tasks while giving us new ideas and more time to live fulfilling lives. It would be great to start with daily activities such as doing dishes and bringing in groceries with drones, or it would be even nicer to have self-charging devices for a start :)
Conclusion
In this post, I did some Python coding with chatGPT. We have coded a neuron, a simple neural network, and learned how to train it. I am pleased with the result. I think that chatGPT has excellent potential for CS students and all coders that want to update their skills effectively. Is it an end of the StackOverflow? We cannot see the feature. However, we can start dreaming about a more exciting and fulfilling lifestyle wherein AI helps us with tedious and boring tasks. We will always need social interaction with humans, and AI bots cannot substitute human communication. Please let me know your ideas or suggestions about this post; your input is always welcome.
Did you like this post? Please let me know if you have any comments or suggestions.
Posts about AI that might be interesting for youGet Updates on AI and Python Coding
Subscribe to my 5-minute newsletter & Get a FREE Git Cheatsheet with Contribution Workflow!
I am committed to your privacy. The information you provide is used to contact you about our relevant content and services. You may unsubscribe from these communications at any time. For more information, write me.
References
1. New Chat (chatGPT by OpenAI)
![]() |
About Elena Elena, a PhD in Computer Science, simplifies AI concepts and helps you use machine learning.
|